Fungible tokens on Aptos
A summary of fungible tokens on Aptos with links to key resources
Overview
This doc provides an overview of the different types of fungible tokens on the Aptos blockchain. It's meant for both non-technical and technical audiences - giving an overview and then linking to more code examples and resources for developers who want to learn more. To learn about non-fungible tokens (NFTs) on Aptos, see our guide.
Terms: Often, the native currency of a chain is referred to as a coin (e.g. APT for the Aptos blockchain), and currencies that live on that chain are referred to as tokens (e.g. the CELL token we reference below). But you'll also hear both referred to as a coin, token, or fungible asset at times. To pick a general term that applies to all cases, we're calling them fungible tokens here.
Types of tokens on APTOS
APT
The native currency of the Aptos blockchain. Used to pay the gas fees for transactions and often used to pay for other things like NFTs - including this interesting-looking creature listed for 3.68 APT:
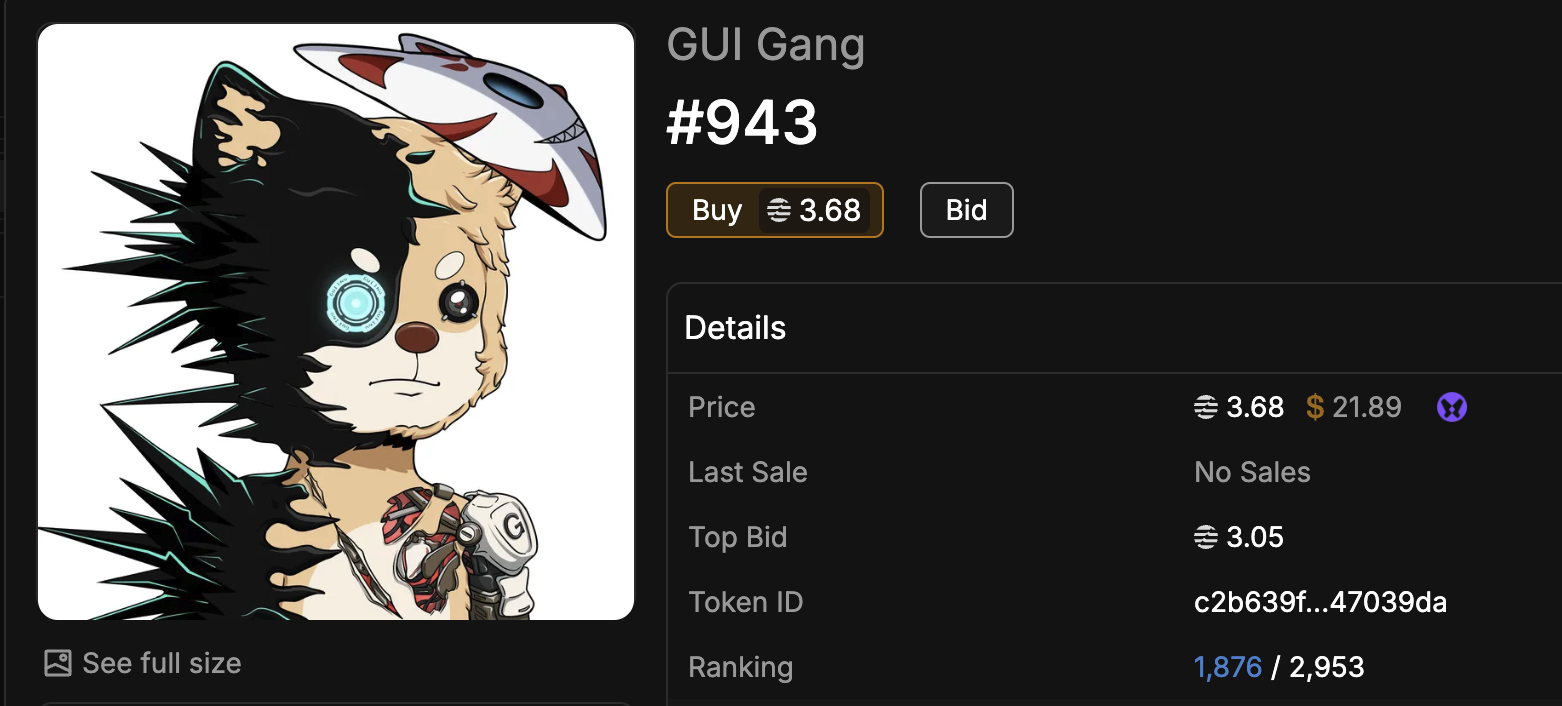
In addition to buying assets and paying for gas, you can stake your APT with a validator, helping to secure the network in exchange for staking rewards. Aptos is a delegated proof-of-stake blockchain, meaning value in the form of staked APT secures the network (unlike Bitcoin, which is proof of work, meaning value in the form of computing resources secures the network).
Like the tokens of other blockchains, the price of APT can vary a lot over time. This variance means that gas and NFT prices can fluctuate day-to-day in fiat terms. Not all tokens on Aptos have this variance, as you'll see in the next section.
Stablecoins
Stablecoins are tokens that are pegged to an underlying asset, often a fiat currency like the US dollar. They are an effort to put value on chain without the price fluctuations that tokens like APT experience. This provides safer ways to perform certain financial transactions, combining the speed and low fees of the Aptos blockchain with the relative stability of certain fiat currencies. For example, international remittance payments and a coffee shop taking payment for coffee both benefit from knowing that the value transmitted one day won't be worth, for example, 5% less the next day.
Stablecoins can be bridged from another chain (like USDC bridged from the Ethereum blockchain) or native to Aptos (like native USDC). Native stablecoins are generally safer because they don't have the additional trust assumptions that a bridge has (you have to trust the code and the team behind the bridge, in addition to the team and the code behind the stable coin). Native stablecoins on Aptos include Circle's USDC and Tether's USDT.
Other fungible tokens
This guide covers the current Fungible Asset Standard and not the legacy Aptos Coin Standard. The new standard provides a consistent way to represent currencies, real-world-assets (e.g. stock shares), and other fungible assets. This standardization means that all apps - explorers, wallets, marketplaces, DeFi apps, etc - can read use these assets in the same way.
It's fairly common for apps to have a token as a way to provide token holders with governance power to help shape the future of the app, as well as financial/reward incentives (for example Cellana's CELL token, here on CoinGecko, which allows users some control over the protocol). In addition to tokens created by apps, there is also the realm of memecoins - e.g. Uptos. This is not a plug for any token or financial advice, just examples of the types of fungible tokens you'll see. Apps like Move Pump have made it easy for non-developers to create tokens as well. You can see a list of all the coins on Aptos here.
Creating and using fungible tokens
As described in the Fungible Asset Standard guide , this involves three steps:
- Create a non-deletable Object to own the new fungible assets metadata. The creator of the asset-type owns this object, and it holds information like the name, symbol, and number of decimal places of the asset. It can't be deleted because if accounts own the asset then losing this general information about the asset type would be catastrophic.
- Generate
Ref
s to enable any desired permissions (e.g.MintRef
for the capability to mint the asset,TransferRef
for the capability to freeze and unfreeze the transfer rights of a given account - important for meeting certain regulations - andBurnRef
for the capability to burn the asset). - Mint the assets and transfer them to accounts.
Steps 1 and 2 are shown below. This is a partial example taken from a larger Aptos Labs example.
module FACoin::fa_coin {
use aptos_framework::fungible_asset::{Self, MintRef, TransferRef, BurnRef, Metadata, FungibleAsset};
use aptos_framework::object::{Self, Object};
use aptos_framework::primary_fungible_store;
use aptos_framework::function_info;
use aptos_framework::dispatchable_fungible_asset;
use std::error;
use std::signer;
use std::string::{Self, utf8};
use std::option;
const ASSET_SYMBOL: vector<u8> = b"FA";
#[resource_group_member(group = aptos_framework::object::ObjectGroup)]
/// Hold refs to control the minting, transfer and burning of fungible assets.
struct ManagedFungibleAsset has key {
mint_ref: MintRef,
transfer_ref: TransferRef,
burn_ref: BurnRef,
}
/// Initialize metadata object and store the refs.
// :!:>initialize
fun init_module(admin: &signer) {
let constructor_ref = &object::create_named_object(admin, ASSET_SYMBOL);
primary_fungible_store::create_primary_store_enabled_fungible_asset(
constructor_ref,
option::none(),
utf8(b"FA Coin"), /* name */
utf8(ASSET_SYMBOL), /* symbol */
8, /* decimals */
utf8(b"http://example.com/favicon.ico"), /* icon */
utf8(b"http://example.com"), /* project */
);
// Create mint/burn/transfer refs to allow creator to manage the fungible asset.
let mint_ref = fungible_asset::generate_mint_ref(constructor_ref);
let burn_ref = fungible_asset::generate_burn_ref(constructor_ref);
let transfer_ref = fungible_asset::generate_transfer_ref(constructor_ref);
let metadata_object_signer = object::generate_signer(constructor_ref);
move_to(
&metadata_object_signer,
ManagedFungibleAsset { mint_ref, transfer_ref, burn_ref }
); // <:!:initialize
// more module code
Creating and using Stablecoins
Aptos Labs has good sample code for creating a stablecoin so check that out if you want to make one.
If you want some Testnet USDC for testing a stablecoin in your transactions and Move code, see Circle's Testnet faucet.
Reading token data to use in your app
Example account
For the following examples, we're going to fetch data for this Testnet account that has three fungible token types
0x1::aptos_coin::AptosCoin
0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake
0x69091fbab5f7d635ee7ac5098cf0c1efbe31d68fec0f2cd565e8d168daf52832
(Testnet USDC)
and one non-fungible asset (an NFT sword for a pretend game).
REST API
Coin balances for an account
You can make a `GET / accounts/{address}/balance/{asset_type} request for the balance of a specific asset type. A request for 0x1::aptos_coin::AptosCoin
is shown - using both cURL and our TypeScript SDK but you can make one for the other two fungible asset types of the account (shown above) as well.
Request
curl --request GET \
--url https://api.shinami.com/aptos/node/v1/accounts/0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de/balance/0x1::aptos_coin::AptosCoin \
--header 'Authorization: Bearer SHINAMI_TESTNET_NODE_API_KEY' \
--header 'accept: application/json' | json_pp
import { createAptosClient } from "@shinami/clients/aptos";
const shinamiAptosClient = createAptosClient(SHINAMI_TESTNET_NODE_ACCESS_KEY);
await aptosClient.getAccountCoinAmount({
accountAddress: "0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de",
coinType: "0x1::aptos_coin::AptosCoin"
});
Response
400000400
# 4.00000400 APT
400000400
// 4.00000400 APT
GraphQL Indexer API
Coin balances for an account along with coin and metadata
You can use the getAccountCoinsData
from our TypeScript SDK or construct a current_fungible_asset_balances
query directly as shown below.
Request
import { createAptosClient } from "@shinami/clients/aptos";
const shinamiAptosClient = createAptosClient(SHINAMI_TESTNET_NODE_ACCESS_KEY);
await aptosClient.getAccountCoinsData({
accountAddress: "0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de"
});
curl -X POST https://api.shinami.com/aptos/graphql/v1 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer SHINAMI_TESTNET_NODE_API_KEY" \
-d '{
"query":"query MyQuery {current_fungible_asset_balances(where: {owner_address: {_eq: \"0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de\"}, token_standard: {}}) { amount_v1 amount_v2 asset_type_v1 asset_type_v2 last_transaction_timestamp last_transaction_timestamp_v1 last_transaction_timestamp_v2 last_transaction_version last_transaction_version_v1 last_transaction_version_v2 metadata { token_standard symbol supply_aggregator_table_key_v1 supply_aggregator_table_handle_v1 project_uri name last_transaction_version last_transaction_timestamp icon_uri decimals creator_address asset_type}}}"
}' | json_pp
Response
[
{
amount: 400000400,
asset_type: '0x1::aptos_coin::AptosCoin',
is_frozen: false,
is_primary: true,
last_transaction_timestamp: '2024-07-08T20:59:37',
last_transaction_version: 4401107226,
owner_address: '0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de',
storage_id: '0x0f34dbbf79be72a2eacc2da1b605327ec89d50531d8a2a5046d7e2cc362a7897',
token_standard: 'v1',
metadata: {
token_standard: 'v1',
symbol: 'APT',
supply_aggregator_table_key_v1: '0x619dc29a0aac8fa146714058e8dd6d2d0f3bdf5f6331907bf91f3acd81e6935',
supply_aggregator_table_handle_v1: '0x1b854694ae746cdbd8d44186ca4929b2b337df21d1c74633be19b2710552fdca',
project_uri: null,
name: 'Aptos Coin',
last_transaction_version: 0,
last_transaction_timestamp: '1970-01-01T00:00:00',
icon_uri: null,
decimals: 8,
creator_address: '0x0000000000000000000000000000000000000000000000000000000000000001',
asset_type: '0x1::aptos_coin::AptosCoin'
}
},
{
amount: 10000000,
asset_type: '0x69091fbab5f7d635ee7ac5098cf0c1efbe31d68fec0f2cd565e8d168daf52832',
is_frozen: false,
is_primary: true,
last_transaction_timestamp: '2025-03-05T18:14:01',
last_transaction_version: 6644150380,
owner_address: '0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de',
storage_id: '0xb665e50deac9b3fe8a1a62e3a6a82f3dd6ac059d561bd64e181025aa42114082',
token_standard: 'v2',
metadata: {
token_standard: 'v2',
symbol: 'USDC',
supply_aggregator_table_key_v1: null,
supply_aggregator_table_handle_v1: null,
project_uri: 'https://circle.com/usdc',
name: 'USDC',
last_transaction_version: 6643331561,
last_transaction_timestamp: '2025-03-04T18:15:06',
icon_uri: 'https://circle.com/usdc-icon',
decimals: 6,
creator_address: '0x72d1e6aa6a648a3afc5d45d8d66b353f1e1837a728c0813beec77f28a697fa7a',
asset_type: '0x69091fbab5f7d635ee7ac5098cf0c1efbe31d68fec0f2cd565e8d168daf52832'
}
},
{
amount: 21674,
asset_type: '0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake',
is_frozen: false,
is_primary: true,
last_transaction_timestamp: '2025-03-04T21:31:22',
last_transaction_version: 6643448594,
owner_address: '0x006c55632dca03fe10c78f5e983cfd560ec55e587d930aa12ea50d75ea3262de',
storage_id: '0x368a1f18aaad1324a8e5293a6f961ca75438e268f2e14c51aa3abc7dc8e40b3e',
token_standard: 'v1',
metadata: {
token_standard: 'v1',
symbol: 'Cake',
supply_aggregator_table_key_v1: null,
supply_aggregator_table_handle_v1: null,
project_uri: null,
name: 'PancakeSwap Token',
last_transaction_version: 2689373781,
last_transaction_timestamp: '2024-07-02T06:57:30',
icon_uri: null,
decimals: 8,
creator_address: '0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf',
asset_type: '0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake'
}
}
]
{
"data" : {
"current_fungible_asset_balances" : [
{
"amount_v1" : 400000400,
"amount_v2" : null,
"asset_type_v1" : "0x1::aptos_coin::AptosCoin",
"asset_type_v2" : null,
"last_transaction_timestamp" : "2024-07-08T20:59:37",
"last_transaction_timestamp_v1" : "2024-07-08T20:59:37",
"last_transaction_timestamp_v2" : null,
"last_transaction_version" : 4401107226,
"last_transaction_version_v1" : 4401107226,
"last_transaction_version_v2" : null,
"metadata" : {
"asset_type" : "0x1::aptos_coin::AptosCoin",
"creator_address" : "0x0000000000000000000000000000000000000000000000000000000000000001",
"decimals" : 8,
"icon_uri" : null,
"last_transaction_timestamp" : "1970-01-01T00:00:00",
"last_transaction_version" : 0,
"name" : "Aptos Coin",
"project_uri" : null,
"supply_aggregator_table_handle_v1" : "0x1b854694ae746cdbd8d44186ca4929b2b337df21d1c74633be19b2710552fdca",
"supply_aggregator_table_key_v1" : "0x619dc29a0aac8fa146714058e8dd6d2d0f3bdf5f6331907bf91f3acd81e6935",
"symbol" : "APT",
"token_standard" : "v1"
}
},
{
"amount_v1" : null,
"amount_v2" : 10000000,
"asset_type_v1" : null,
"asset_type_v2" : "0x69091fbab5f7d635ee7ac5098cf0c1efbe31d68fec0f2cd565e8d168daf52832",
"last_transaction_timestamp" : "2025-03-05T18:14:01",
"last_transaction_timestamp_v1" : null,
"last_transaction_timestamp_v2" : "2025-03-05T18:14:01",
"last_transaction_version" : 6644150380,
"last_transaction_version_v1" : null,
"last_transaction_version_v2" : 6644150380,
"metadata" : {
"asset_type" : "0x69091fbab5f7d635ee7ac5098cf0c1efbe31d68fec0f2cd565e8d168daf52832",
"creator_address" : "0x72d1e6aa6a648a3afc5d45d8d66b353f1e1837a728c0813beec77f28a697fa7a",
"decimals" : 6,
"icon_uri" : "https://circle.com/usdc-icon",
"last_transaction_timestamp" : "2025-03-04T18:15:06",
"last_transaction_version" : 6643331561,
"name" : "USDC",
"project_uri" : "https://circle.com/usdc",
"supply_aggregator_table_handle_v1" : null,
"supply_aggregator_table_key_v1" : null,
"symbol" : "USDC",
"token_standard" : "v2"
}
},
{
"amount_v1" : 21674,
"amount_v2" : null,
"asset_type_v1" : "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake",
"asset_type_v2" : null,
"last_transaction_timestamp" : "2025-03-04T21:31:22",
"last_transaction_timestamp_v1" : "2025-03-04T21:31:22",
"last_transaction_timestamp_v2" : null,
"last_transaction_version" : 6643448594,
"last_transaction_version_v1" : 6643448594,
"last_transaction_version_v2" : null,
"metadata" : {
"asset_type" : "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake",
"creator_address" : "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf",
"decimals" : 8,
"icon_uri" : null,
"last_transaction_timestamp" : "2024-07-02T06:57:30",
"last_transaction_version" : 2689373781,
"name" : "PancakeSwap Token",
"project_uri" : null,
"supply_aggregator_table_handle_v1" : null,
"supply_aggregator_table_key_v1" : null,
"symbol" : "Cake",
"token_standard" : "v1"
}
}
]
}
}
Metadata for a coin
You can use the getFungibleAssetMetadata
from our TypeScript SDK or construct a fungible_asset_metadata
query directly as shown below.
Request
import { createAptosClient } from "@shinami/clients/aptos";
const shinamiAptosClient = createAptosClient(SHINAMI_TESTNET_NODE_ACCESS_KEY);
await aptosClient.getFungibleAssetMetadata({
minimumLedgerVersion: undefined,
options: {
where: {
asset_type: {
_eq: "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake"
}
}
}
});
curl -X POST https://api.shinami.com/aptos/graphql/v1 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer SHINAMI_TESTNET_NODE_API_KEY" \
-d '{
"query": "query MyQuery { fungible_asset_metadata(where: {asset_type: {_eq: \"0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake\"}}) { icon_uri maximum_v2 project_uri supply_aggregator_table_handle_v1 supply_aggregator_table_key_v1 supply_v2 symbol token_standard name last_transaction_version last_transaction_timestamp decimals creator_address asset_type}}"
}' | json_pp
Response
[
{
icon_uri: null,
project_uri: null,
supply_aggregator_table_handle_v1: null,
supply_aggregator_table_key_v1: null,
creator_address: '0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf',
asset_type: '0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake',
decimals: 8,
last_transaction_timestamp: '2024-07-02T06:57:30',
last_transaction_version: 2689373781,
name: 'PancakeSwap Token',
symbol: 'Cake',
token_standard: 'v1',
supply_v2: null,
maximum_v2: null
}
]
{
"data" : {
"fungible_asset_metadata" : [
{
"asset_type" : "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf::pancake::Cake",
"creator_address" : "0xe0e5ad285cbcdb873b2ee15bb6bcac73d9d763bcb58395e894255eeecf3992cf",
"decimals" : 8,
"icon_uri" : null,
"last_transaction_timestamp" : "2024-07-02T06:57:30",
"last_transaction_version" : 2689373781,
"maximum_v2" : null,
"name" : "PancakeSwap Token",
"project_uri" : null,
"supply_aggregator_table_handle_v1" : null,
"supply_aggregator_table_key_v1" : null,
"supply_v2" : null,
"symbol" : "Cake",
"token_standard" : "v1"
}
]
}
}
Resources
Here, we list many of the resources we listed above in a compact form:
General
- Fungible tokens on Aptos by marketcap (including APT and stablecoins)
APT
- Transaction fee calculation (gas and storage fees).
- Delegated staking
Other fungible tokens
Stablecoins
- How to build a stablecoin on Aptos sample code.
- Stablecoins on Aptos by market cap.
Updated 11 days ago