Node Service: send and debug a request
Send a request. See the error in your Shinami dashboard. Look up what to do in our Error Guide.
You'll learn how to
- Send a request to Aptos Node Service.
- View your requests and error codes in your Shinami dashboard
- Get guidance on a specific error code in our Error guide.
Steps
1. Create a Shinami account
You’ll need a Shinami account to follow this quick-start guide.
2. Create an API Access Key
To use Shinami's products, you need an API access key to authenticate your requests. Set up a Node Access key for Testnet as shown here in our Authentication and API Keys guide
3. Make a request!
Here is an example sui_getTransactionBlock
request that returns data about a given transaction digest.
Example Request with an error
Replace all instances of {{name}} with the actual value for that name. The TypeScript example uses our Shinami Clients SDK.
curl https://api.shinami.com/sui/node/v1 \
-X POST \
-H 'X-API-Key: {{nodeAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc":"2.0",
"method":"sui_getTransactionBlock",
"params":[
"22222222222222222222222222222222222222222222",
{
"showInput": true,
"showRawInput": true,
"showEffects": true,
"showEvents": true,
"showObjectChanges": true,
"showBalanceChanges": true
}
],
"id":1
}' | json_pp
import { createSuiClient } from "@shinami/clients/sui";
const nodeClient = createSuiClient({{nodeAccessKey}});
const resp = await nodeClient.getTransactionBlock({
digest: "22222222222222222222222222222222222222222222",
options: {
showEvents: true,
showBalanceChanges: true,
showInput: true,
showRawInput: true,
showObjectChanges: true,
showEffects: true
}
});
console.log(resp);
Error Response
{
"error" : {
"code" : -32602,
"message" : "Could not find the referenced transaction [TransactionDigest(22222222222222222222222222222222222222222222)]."
},
"id" : 1,
"jsonrpc" : "2.0"
}
JsonRpcError: Could not find the referenced transaction [TransactionDigest(22222222222222222222222222222222222222222222)].
at ... {
code: -32602,
type: 'InvalidParams'
}
4. View the request and error in your dashboard
On the Sui Node Service page of your dashboard, you can view a rich set of API insights. It can take a few minutes for a request to show up. If your request hasn't shown up yet, just keep reading this doc to learn about the in-dashboard metrics and error guide, then refresh the your dashboard page.
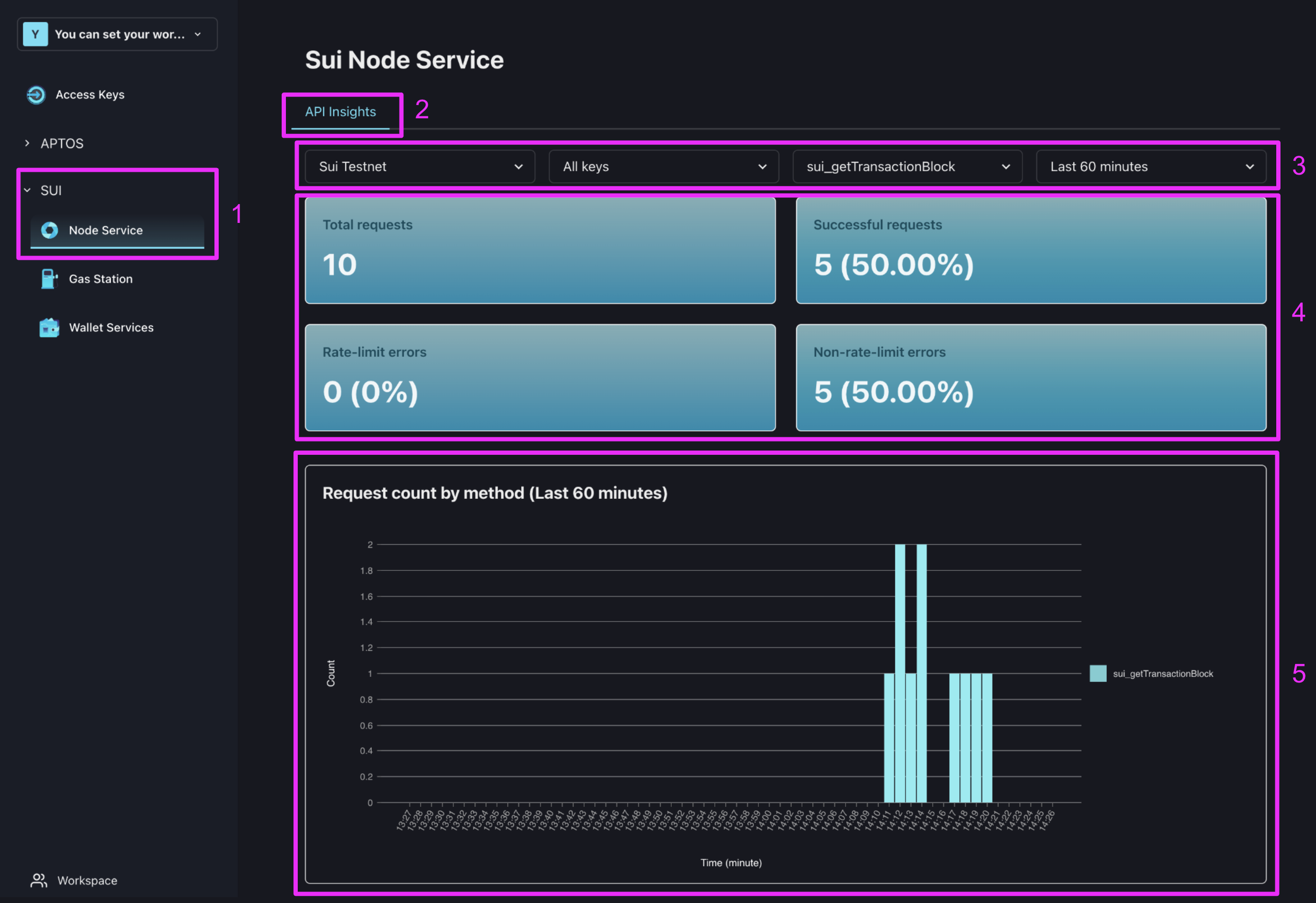
- Each service has its own page with its own API insights.
- Sui Node Service has only one tab, but others have more.
- Filters: I've chosen "Sui Testnet", "sui_getTransactionBlock", and "Last 60 minutes" to narrow down to this one request. It's often good to look at errors across all methods and then drill down into individual methods.
- Summary metrics: a great place to get a quick overview of your integration health. If there's an issue - like a lot of rate-limit or other errors, you can scroll down to the graphs below to see when they occurred and what the error codes were. When you filter by an individual method or API key or network, these update to reflect that.
- Graphs: this is the first one, showing requests by method name. I'll only see "sui_getTransactionBlock", since I'm filtering by that method. I sent a few examples when preparing this guide. Your graph will show as many as you sent.
Scroll down to view more graphs, including a breakdown of errors by JSON-RPC error code. Not all my requests failed, which is why this graph isn't as populated as the first one. Recall that I've filtered my graphs to show only "sui_getTransactionBlock" requests. Other requests of mine may have gotten errors - including -32602
errors - but they won't be shown here. I'd need to change the filter back to the default of "All requests" to see those.
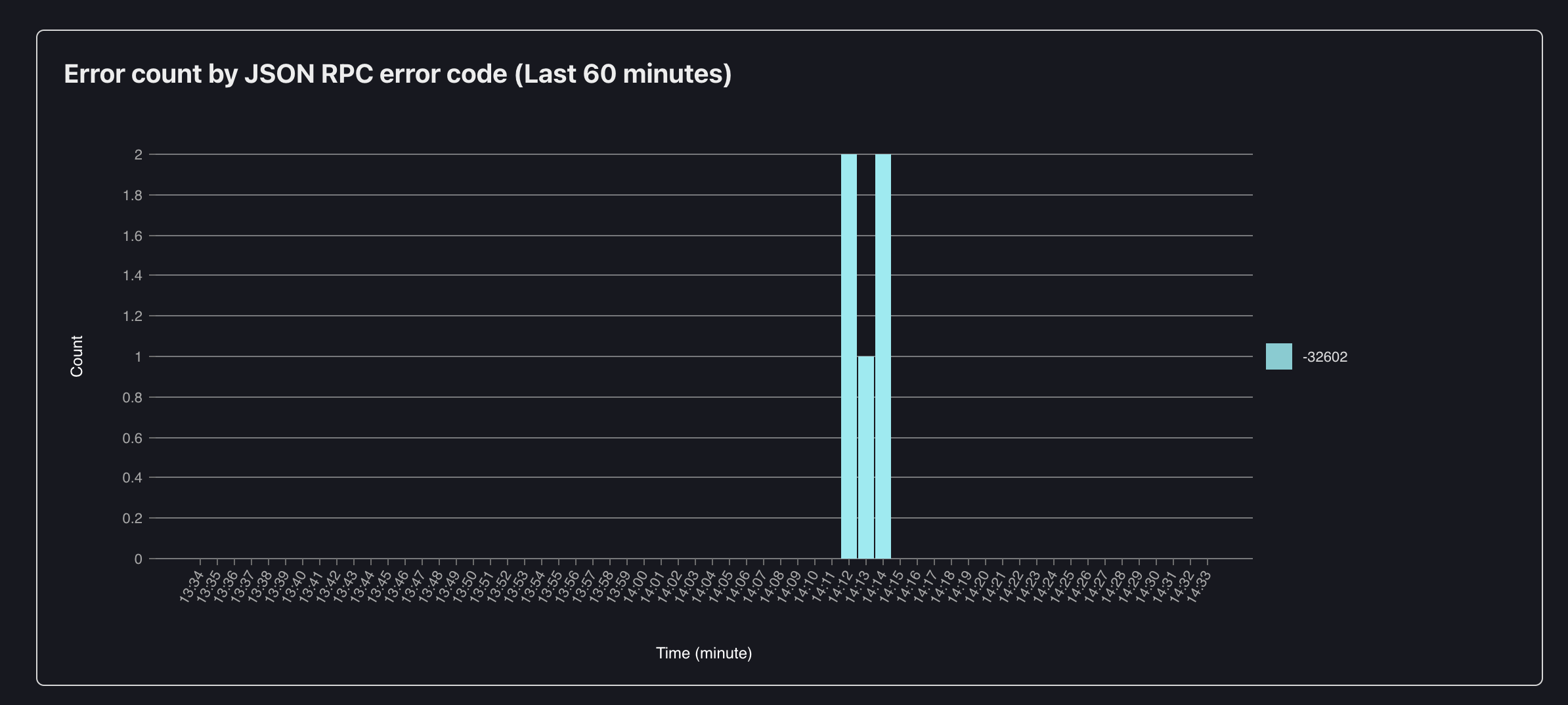
5. See what the error means in our Error guide
Our Error guide has an overall section for all Shinami Services, as well as a specific section for each service, including our Sui Node Service. In this case, we'll find JSON-RPC error -32602: Invalid Params
. The notes tell us, among other things, that on a sui_getTransactionBlock
request it can be because a digest doesn't exist. That's our issue here. We're also told to check the general section above. That's good advice: always check the service-specific section and the general JSON-RPC and HTTP error code sections at the top.
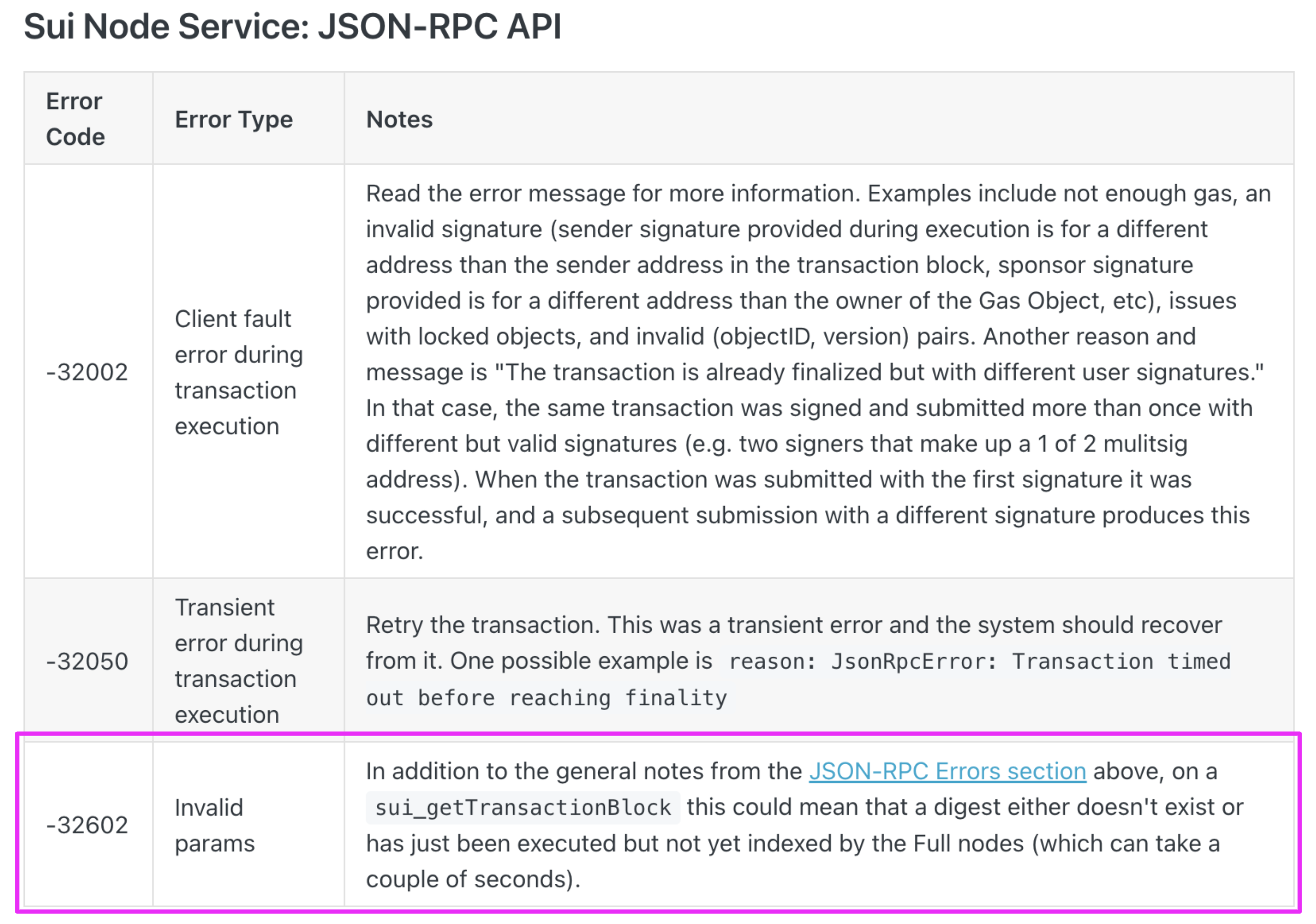
6. Send a successful request
Let's input a valid Testnet digest and try again.
Updated request
Replace all instances of {{name}} with the actual value for that name. The TypeScript example uses our Shinami Clients SDK. Note that for a smaller example response we only set showBalanceChanges
to true
. In your app you'll ask for whatever you need.
curl https://api.shinami.com/sui/node/v1 \
-X POST \
-H 'X-API-Key: {{nodeAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc":"2.0",
"method":"sui_getTransactionBlock",
"params":[
"2zDvVpTkkQJYaW95HNdvBQm8CiSr8ateuoFK57xbRyQ9",
{
"showInput": false,
"showRawInput": false,
"showEffects": false,
"showEvents": false,
"showObjectChanges": false,
"showBalanceChanges": true
}
],
"id":1
}' | json_pp
import { createSuiClient } from "@shinami/clients/sui";
const nodeClient = createSuiClient({{nodeAccessKey}});
const resp = await shinamiTestnetNodeClient.getTransactionBlock({
digest: "2zDvVpTkkQJYaW95HNdvBQm8CiSr8ateuoFK57xbRyQ9",
options: {
showEvents: false,
showBalanceChanges: true,
showInput: false,
showRawInput: false,
showObjectChanges: false,
showEffects: false
}
});
console.log(resp);
Successful response
{
"id" : 1,
"jsonrpc" : "2.0",
"result" : {
"balanceChanges" : [
{
"amount" : "-1028196",
"coinType" : "0x2::sui::SUI",
"owner" : {
"AddressOwner" : "0xfc837a36850adfd5ea076de8c1638ad7c2d228c91d9fe3e2771ff305cb52c07c"
}
}
],
"checkpoint" : "125146246",
"digest" : "2zDvVpTkkQJYaW95HNdvBQm8CiSr8ateuoFK57xbRyQ9",
"timestampMs" : "1730149869581"
}
}
{
digest: '2zDvVpTkkQJYaW95HNdvBQm8CiSr8ateuoFK57xbRyQ9',
balanceChanges: [
{ owner: [Object], coinType: '0x2::sui::SUI', amount: '-1028196' }
],
timestampMs: '1730149869581',
checkpoint: '125146246'
}
Next step: build on Sui! 🏗
- For an overview of our API docs and tutorials for each of our services, see our API References Overview.
- For tips on learning how to code in Sui Move, see Sui Move Resources
- Check out the Sui Node Service product usage FAQ and billing FAQ in our Help Center for answers to common questions
- If you ever have questions, reach out to us.
Updated 16 days ago