A GraphQL interface to read state from the Aptos Blockchain.
You'll find key usage notes below. If you ever need help you can reach out to us.
Authentication, Rate Limits, and Error Handling
Authentication
Our service URL is https://api.shinami.com/aptos/graphql/v1
. You authenticate via an access key passed in a header ("X-Api-Key: ACCESS_KEY"
or "Authorization: Bearer ACCESS_KEY"
). These steps are done automatically by our TypeScript SDK. For more information, including how to set up API access keys, see our Authentication and API Keys guide.
Rate Limits
When you surpass the CUPS (Compute Units Per Second) limit for a key, we return a HTTP 429. We recommend implementing retries with a backoff to handle any rate limits errors that arise. You can also adjust the rate limits of your keys to better balance your CUPS allotment across your keys. For more on CUPS and monitoring your CU usage, see the Aptos Node Service FAQ in our Help Center.
Error Handling
See our Error Reference guide.
How to Send a Request
When sending a request to our Node Service, make sure to do the following two things (done automatically by our TypeScript SDK).
- Set the base URL to
https://api.shinami.com/aptos/graphql/v1
- Send your access key in one of these two headers:
"Authorization: Bearer YOUR_ACCESS_KEY"
"X-Api-Key: YOUR_ACCESS_KEY"
- Use an access key tied to the network you want to interact with. When you create an API access key in your Shinami dashboard, you give it rights to exactly one network. We route to Mainnet or Testnet based on the API access key you send in the request.
Sample Request
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
curl -X POST https://api.shinami.com/aptos/graphql/v1 \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_ACCESS_KEY" \
-d '{
"query": "query MyQuery { ledger_infos { chain_id } }"
}'
import { createAptosClient } from "@shinami/clients/aptos";
const shinamiAptosClient = createAptosClient(SHINAMI_NODE_ACCESS_KEY);
await aptosClient.queryIndexer({
query: {
query:
`query MyQuery {
ledger_infos {
chain_id
}
}
`
}
});
Example Response
{
"data":{
"ledger_infos":[
{ "chain_id":2 }
]
}
}
{
ledger_infos: [
{ chain_id: 2 }
]
}
Available tables and fields
To see the available tables and fields we support querying, and to create and run sample queries, you can use Hasura's graphqurl. Follow the installation instruction steps in their README. Then you can launch the explorer locally by running:
gq -i https://api.shinami.com/aptos/graphql/v1 \
-H "Authorization: Bearer YOUR_APTOS_NODE_API_KEY"
In your browser, you can visit http://localhost:4500/
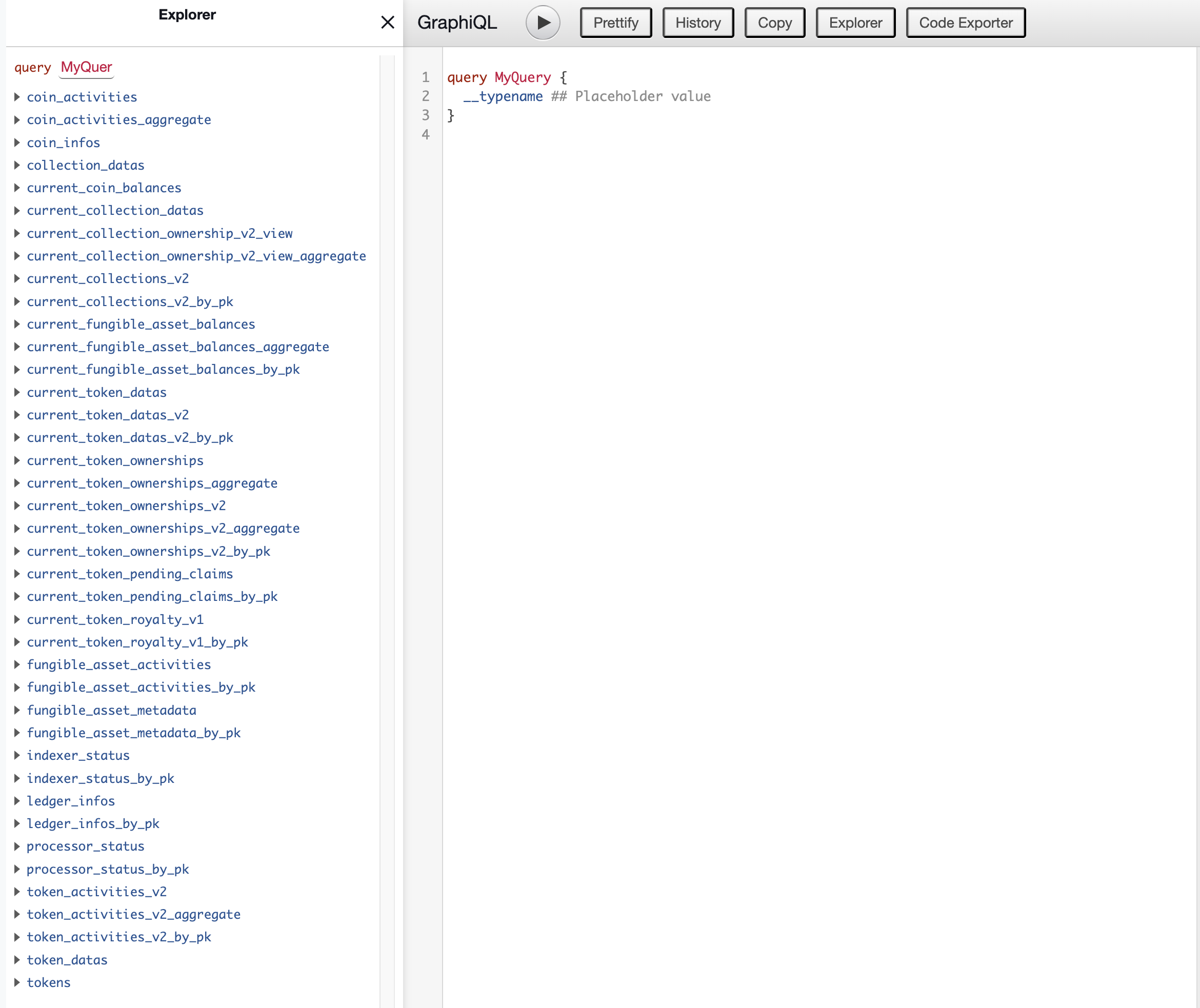
Since you're using your API key, any queries you run here will show up in your API insights (and will count against your CU and CUPS limits).
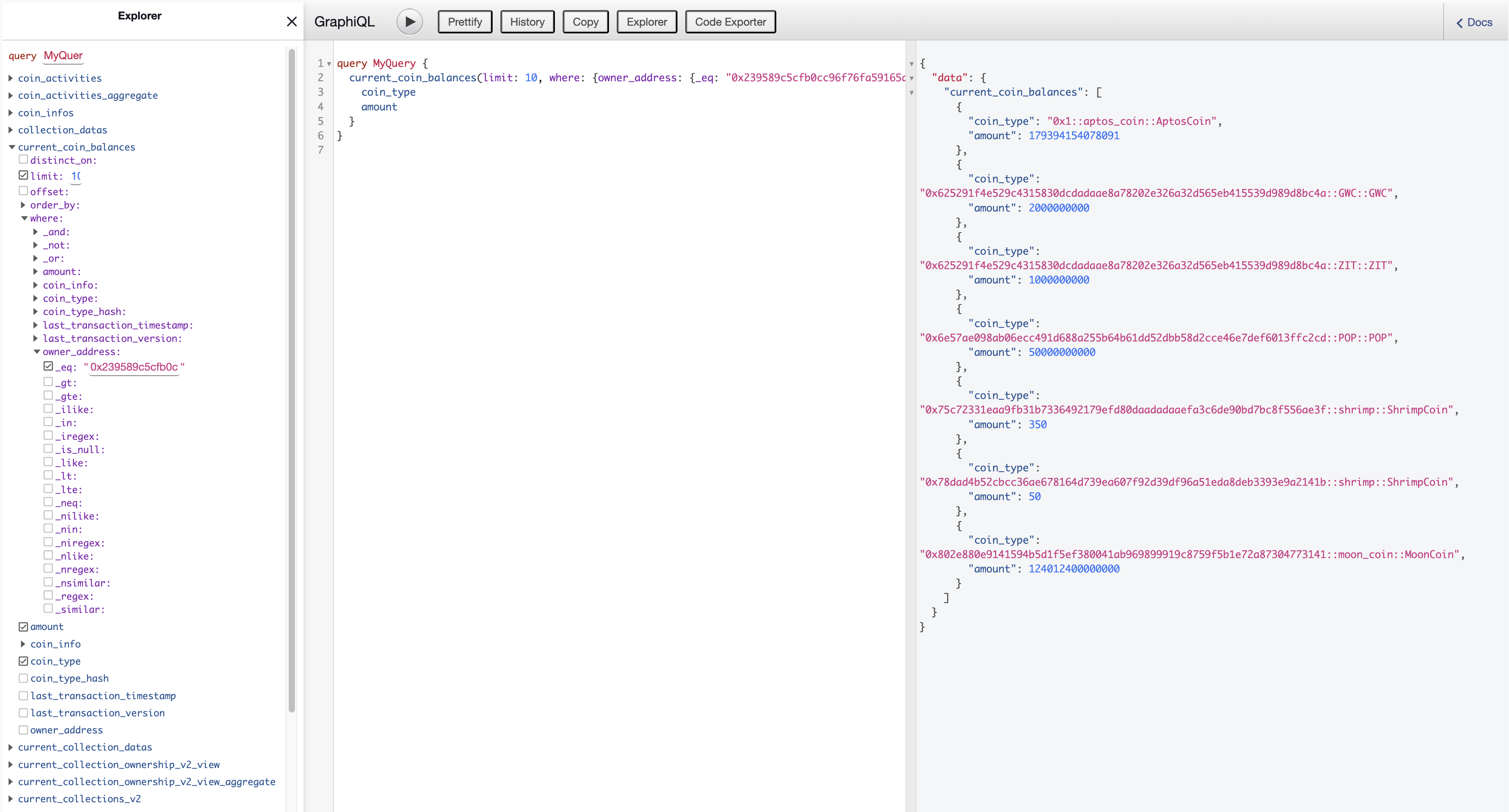