App-controlled embedded wallets for a smooth UX
Overview
You'll find API endpoints and key usage notes below. If you ever need help you can reach out to us.
Use Cases
Shinami’s Invisible Wallets abstract away Web3 elements like seed phrases, third-party wallet connections, gas fees, and signing popups. They are embedded, backend wallets under the shared custody of your app and Shinami. Both parties must cooperate in order to obtain a valid signature.
We also offer user-controlled zkLogin wallet services.
Shinami Gas Station Integration
All methods below that write to the Sui blockchain have their gas fees sponsored by you via a Gas Station you create (see the Sui Gas Station FAQ page of our Help Center for how guidance on how to set up a fund and add free Testnet Sui to it). This is because Invisible Wallets are designed to easily onboard Web2-native users (who may not want to download a wallet app, manage a seed phrase, and complete KYC checks to buy SUI for gas).
Authentication, Rate Limits and Error Handling
Authentication
You authenticate via an access key passed in a header ('X-Api-Key: ACCESS_KEY') or in the request url, e.g. https://api.shinami.com/sui/wallet/v1/ACCESS_KEY
. We recommend using a request header and not putting access keys in your request URLs for reduced visibility (in logs, etc). These steps are done automatically by our TypeScript SDK.
For more information, including how to set up an access key with Wallet Services rights, see our Authentication and API Keys guide.
Call this API from your backend
Shinami Wallet Services do not support CORS requests, so if you call these APIs from your frontend you'll get a CORS error. This is for security reasons: exposed keys and wallet information could lead to malicious actors signing transactions on behalf of your users.
Rate Limits
When you surpass the QPS limit for a key, we return a JSON-RPC error code -32010
. We recommend implementing retries with a backoff to handle any rate limits errors that arise. You can also adjust the rate limits of your keys to better balance your QPS allotment across your keys.
Error Handling
See our Error Reference for guidance on the errors you may receive from our services, including a section on errors specific to the Invisible Wallet API.
WalletId and Secret Pairing
When you create an Invisible Wallet, you must create, store, link, and never change the following two values:
walletId
: Your internal id for a wallet. When you provide us awalletId
in a method call, it tells us which Invisible Wallet to use. It could be your internaluserId
value, or a new arbitrary and unique value you link to theuserId
.secret
: Your internal secret for a wallet. ThesessionToken
you generate with it is combined with Shinami data to obtain a signature from the associated wallet. Ideally it would be different for each wallet so that if onesecret
is compromised the rest are not.
When you create an Invisible Wallet, you forever link its walletId it to the secret you used:
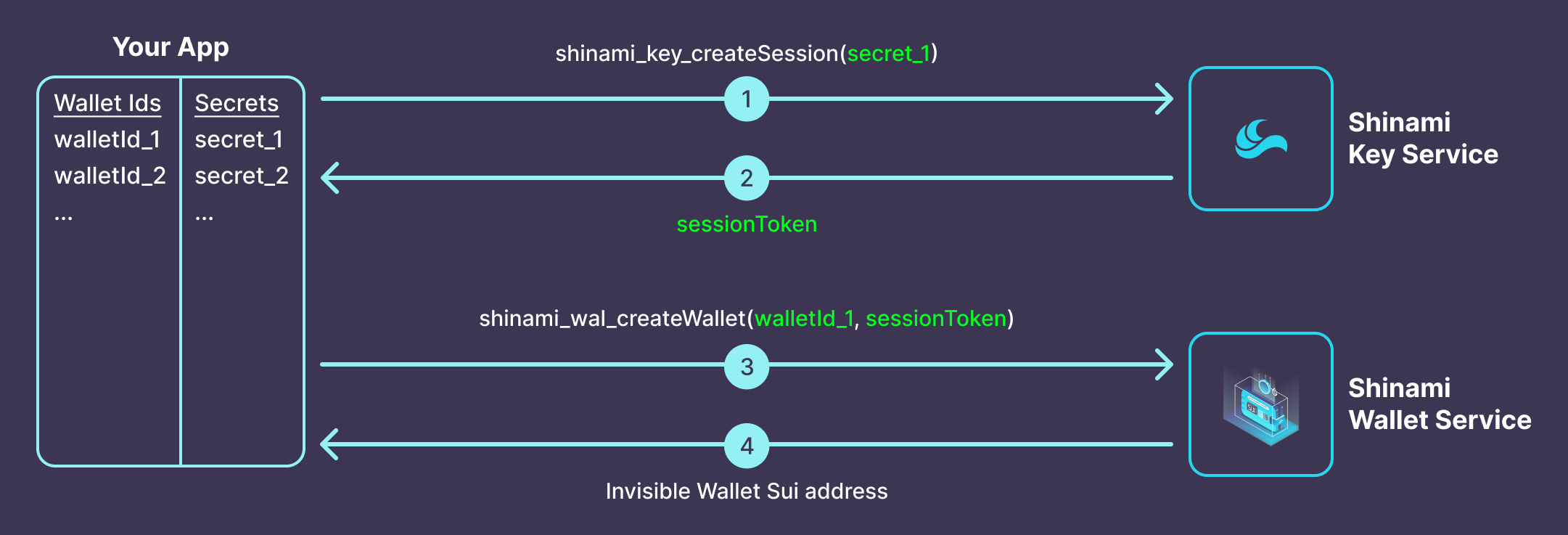
So, if you try to use the walletId with a different secret, you'll get an error:
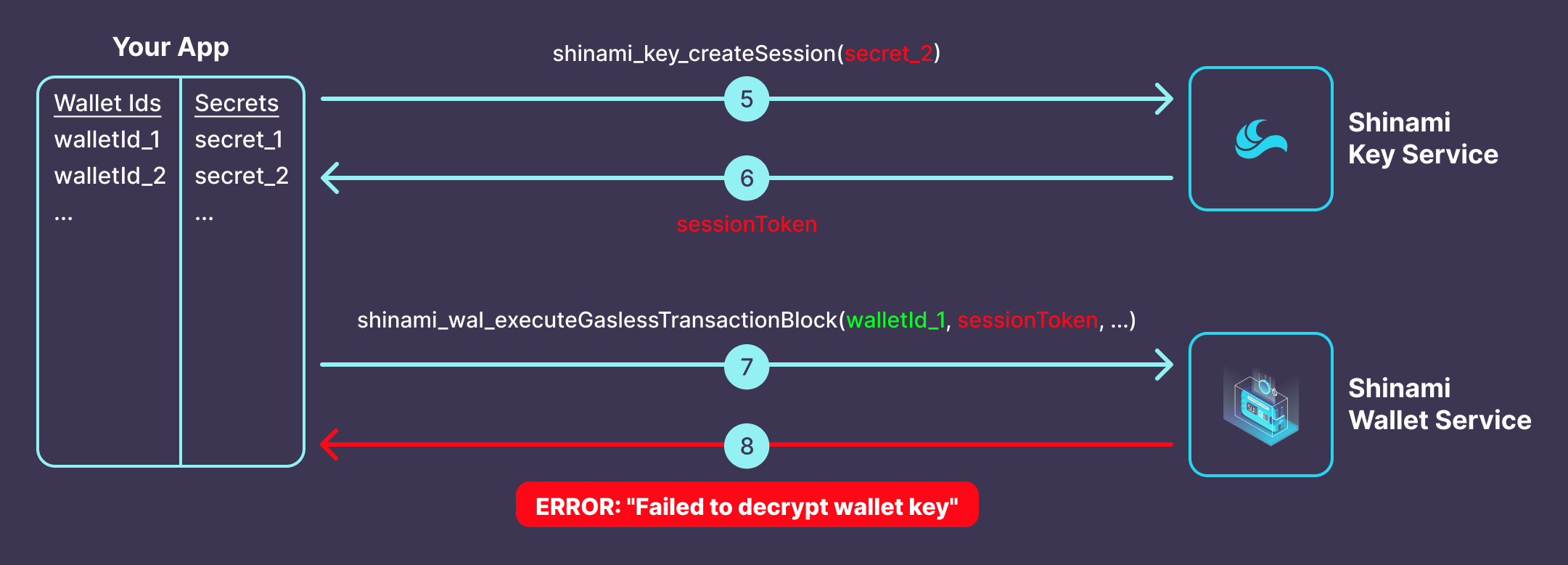
Tutorial with E2E sample code
Check out our TypeScript tutorial for more code samples and details on the end-to-end flow of creating and using Invisible Wallets to execute sponsored transactions.
Methods
shinami_key_createSession
Description
For security purposes, you must generate a session token before you create a wallet, or sign or execute transactions. Session tokens are valid and can be reused for 10 minutes.
You may also use an instance of ShinamiWalletSigner
to manage session token generation and refreshes for a given wallet. This is shown in the methods below that have a sessionToken
parameter in an additional sample code tab.
Request Parameters
Name | Type | Description |
---|---|---|
secret | string | Used to encrypt and decrypt a wallet's private key. Therefore, it must always be used with the same walletId and cannot be changed in the future (see walletId and secret pairing) |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/key/v1 \
-X POST \
-H 'X-API-Key: {{walletAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc":"2.0",
"method":"shinami_key_createSession",
"params":[
"{{secret}}"
],
"id":1
}'
import { KeyClient } from "@shinami/clients/sui";
const key = new KeyClient({{walletAccessKey}});
await key.createSession({{secret}});
Example Response
{
"jsonrpc":"2.0",
"result":"eyJraWQiOiJrZXkyMDIzMDgxMSIsImVuYyI6IkEyNTZHQ00iLCJ0YWciOiI4SVpQWXlHeDlmOTd6U2NIdmN6N3lnIiwiYWxnIjoiQTI1NkdDTUtXIiwiaXYiOiJQWVJXZFJrbnNMMlNnVzhfIn0.ygDCI-NcvUcH7wYc0Bp0-59qeIfGOqLyXZGsLF4pW0M.aOAW0AwBvAWpaS-S.QmesdNIdNIYbT59RET-lNuzNMUvS-xb2exhXrAIlspnIkV3nuBx7PKC_GgJ7C1EqJx3tDtQaLLDGdrO8_s-75oK88ls5mzDRR-w2A0VdCcTH0_JwsQgijIbCKFWS0g.dULMzxZ4gGbm2unqOnzv8w",
"id": 1
}
"eyJraWQiOiJrZXkyMDIzMDgxMSIsImVuYyI6IkEyNTZHQ00iLCJ0YWciOiI4SVpQWXlHeDlmOTd6U2NIdmN6N3lnIiwiYWxnIjoiQTI1NkdDTUtXIiwiaXYiOiJQWVJXZFJrbnNMMlNnVzhfIn0.ygDCI-NcvUcH7wYc0Bp0-59qeIfGOqLyXZGsLF4pW0M.aOAW0AwBvAWpaS-S.QmesdNIdNIYbT59RET-lNuzNMUvS-xb2exhXrAIlspnIkV3nuBx7PKC_GgJ7C1EqJx3tDtQaLLDGdrO8_s-75oK88ls5mzDRR-w2A0VdCcTH0_JwsQgijIbCKFWS0g.dULMzxZ4gGbm2unqOnzv8w"
Response Fields
Name | Type | Description |
---|---|---|
result | string | sessionToken corresponding to the provided secret . Valid and can be reused for 10 minutes. |
shinami_wal_createWallet
Description
Programmatically generates a unique wallet for a user that is Sui network agnostic (has the same address on Devnet, Testnet, and Mainnet). On the free tier you have a limit of wallet creations per month as shown on the "Sui Wallet Services" tab of the billing page in your dashboard (where you can also see how to upgrade if needed). If you hit this limit, you will get a JSON-RPC code -32012
and should not retry. All other wallet operations will still work for the month, like signing with wallets you've already created.
Each
walletId
only works with thesecret
you create it with (via thesessionToken
you pass to this method). Your application MUST remember the (walletId
,secret
) pair associated with each Invisible Wallet you create. If you forget or change either of these values, the wallet's private key will be lost and we cannot recover it for you.
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | A unique ID you maintain for the wallet. Can be based on your internal user IDs. Note: you cannot change this value in the future, so do not use a value that you or your users might change, such as an editable username . |
sessionToken | string | The token generated by shinami_key_createSession with the unalterable secret you will permanently associate with this walletId (and, ideally, only this walletId). |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{walletAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_wal_createWallet",
"params": [
"{{walletId}}",
"{{sessionToken}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{walletAccessKey}});
await walletClient.createWallet(
{{walletId}},
{{sessionToken}}
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
// Returns the Sui address of the invisible wallet,
// creating it if it hasn't been created yet.
const CREATE_WALLET_IF_NOT_FOUND = true;
await signer.getAddress(CREATE_WALLET_IF_NOT_FOUND);
Example Response
{
"jsonrpc":"2.0",
"result":"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f",
"id":1
}
"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f"
"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f"
Response Data
Type | Description |
---|---|
String | The Sui address of the Invisible Wallet created for this walletId. Network-agnostic (the address will be the same on Devnet, Testnet, and Mainnet). |
shinami_wal_getWallet
Description
Retrieve a user's wallet address based your unique walletId value for it.
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet. |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{walletAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc":"2.0",
"method":"shinami_wal_getWallet",
"params":[
"{{walletId}}"
],
"id":1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{walletAccessKey}});
await walletClient.getWallet(
{{walletId}}
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.getAddress();
Example Response
{
"jsonrpc":"2.0",
"result":"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f",
"id":1
}
"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f"
"0xecaeb4a763dd49f2cd13aeaf2e7ab01f704bbc8c2bd9c2e991b726d0632c3b4f"
Response Fields
Type | Description |
---|---|
String | The Sui address of the Invisible Wallet created for this walletId. Network-agnostic (the address will be the same on Devnet, Testnet, and Mainnet). |
shinami_wal_signTransactionBlock
Description
Signs a fully constructed transaction so that it can be executed. This is a low level API - it requires integration with Gas Station API and Sui API for transaction sponsorship (if needed) and execution. This method gives you more control over how you submit transactions to Sui compared to shinami_wal_executeGaslessTransactionBlock
, which sponsors, signs, and executes an Invisible Wallet transaction in one method call.
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet. |
sessionToken | string | The token generated by shinami_key_createSession with the same secret you used when creating this wallet. |
txBytes | SDK: string | Uint8Array cURL: string | BCS serialized TransactionData, which includes gas data. It lacks only the sender's signature (which this method generates) before it can be submitted to the chain. If string , assumed to be Base64 encoded. |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{walletAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_wal_signTransactionBlock",
"params": [
"{{walletId}}",
"{{sessionToken}}",
"{{txBytes}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{walletAccessKey}});
await walletClient.signTransaction(
{{walletId}},
{{sessionToken}},
{{txBytes}}
)
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.signTransaction(
{{txBytes}}
);
Example Response
{
"jsonrpc":"2.0",
"result":{
"signature":"AKzbe4FlhuT9saKFDUEdmCELBVa/NhsERc2XPahGC+8Ar6YMoK+DH+xs8xg/RSYF7HeZ4UmwnSPJFZpYjgWWZQB51Goyfzm4soRhJY9gDmt/wDZYCm81bkCP87eBm1T+Xw==",
"txDigest":"BSFD6oDgftrtcVCZF8EAkUcmWWyd8ZRsMCGSh6EbtqCj"
},
"id":1
}
{
signature: 'AKzbe4FlhuT9saKFDUEdmCELBVa/NhsERc2XPahGC+8Ar6YMoK+DH+xs8xg/RSYF7HeZ4UmwnSPJFZpYjgWWZQB51Goyfzm4soRhJY9gDmt/wDZYCm81bkCP87eBm1T+Xw==',
txDigest: 'BSFD6oDgftrtcVCZF8EAkUcmWWyd8ZRsMCGSh6EbtqCj'
}
{
signature: 'AKzbe4FlhuT9saKFDUEdmCELBVa/NhsERc2XPahGC+8Ar6YMoK+DH+xs8xg/RSYF7HeZ4UmwnSPJFZpYjgWWZQB51Goyfzm4soRhJY9gDmt/wDZYCm81bkCP87eBm1T+Xw==',
txDigest: 'BSFD6oDgftrtcVCZF8EAkUcmWWyd8ZRsMCGSh6EbtqCj'
}
Response Fields
Name | Type | Description |
---|---|---|
signature | string | Base64 encoded transaction signature, signed by the wallet key. To be used alongside the txBytes sent to this method and the gas sponsor's signature (if applicable) in a call to sui_executeTransactionBlock |
txDigest | string | Base 58 encoded transaction digest. |
shinami_wal_signPersonalMessage
Description
Signs a personal message using an Invisible Wallet. The signature can be verified with the PersonalMessage
intent scope. The request template below titled End-to-end example with ShinamiWalletSigner - Shinami TS SDK
shows an end-to-end example of signing and a message and verifying a signature.
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet. |
sessionToken | string | The token generated by shinami_key_createSession with the same secret you used when creating this wallet. |
message | string | Message bytes to be signed. See an example in the request template below titled End-to-end example with ShinamiWalletSigner - Shinami TS SDK |
wrapBcs | boolean | Optional. Set it to true when calling the API directly to match the verification behavior of the Sui TypeScript SDK. When using the Shinami TypeScript SDK it's set to true by default. |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{walletAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_wal_signPersonalMessage",
"params": [
"{{walletId}}",
"{{sessionToken}}",
"{{message}}",
{{wrapBCs}}
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{walletAccessKey}});
await walletClient.signPersonalMessage(
{{walletId}},
{{sessionToken}},
{{message}},
{{wrapBCS}} // optional, defaults to true if not provided
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
import { verifyPersonalMessage } from '@mysten/sui.js/verify';
const walletClient = new WalletClient({{walletAccessKey}});
const key = new KeyClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
// encode the as a base64 string
let message = "I have the private keys.";
let messageAsBase64String = btoa(message);
// use Shinami Wallet Service to sign the message
let signature = await signer.signPersonalMessage(
messageAsBase64String
);
// When we check the signature, we encode the message as a byte array
// and not a base64 string like when we signed it
let messageBytes = new TextEncoder().encode(message);
// Failure throws a `Signature is not valid for the provided message` Error
let publicKey = await verifyPersonalMessage(messageBytes, signature);
// Get the wallet address we signed with so we can check against it
let walletAddress = await signer.getAddress();
console.log(walletAddress == publicKey.toSuiAddress());
Example Response
{
"jsonrpc":"2.0",
"result":"AFKIGo7e/eaqCbrrDKIVh4wjpHVudqP8Pbdzo+spztZGmUfiDPY9EPnTx7RnadSQHCSpxgP+QwaAvsJc4JMfswR51Goyfzm4soRhJY9gDmt/wDZYCm81bkCP87eBm1T+Xw==",
"id":1
}
"AFKIGo7e/eaqCbrrDKIVh4wjpHVudqP8Pbdzo+spztZGmUfiDPY9EPnTx7RnadSQHCSpxgP+QwaAvsJc4JMfswR51Goyfzm4soRhJY9gDmt/wDZYCm81bkCP87eBm1T+Xw=="
true
Response Data
Type | Description |
---|---|
string | Base64 encoded signature, produced by the private key of the Invisible Wallet associated with the provided walletId . |
shinami_wal_executeGaslessTransactionBlock
Description
Sponsors, signs, and executes a gasless transaction from a wallet. This is a convenient end-to-end method for submitting sponsored transactions to the chain when you also use Shinami Gas Station. It sponsors the transaction using the Gas Station fund tied to the access key used to make the request. To see how to set up an Access Key with rights to all services, see our Authentication and API Keys guide. Note that this call produces a Node service sui_executeTransactionBlock
request which counts against your daily request total (and so your billing).
You cannot use the gas object in a sponsored transaction for other purposes: For example, you cannot write const [coin] = txb.splitCoins(txb.gas,[txb.pure(100)]);
because it's accessing txb.gas
. If you try to sponsor a TransactionKind that uses the gas object you will get a JSON-RPC -32602
error back from the Gas Station sponsorship attempt.
Shinami sponsorship fees: We charge a small fee (in SUI) per sponsorship request to cover our costs. For details, visit the Billing tab in your Shinami dashboard.
To call this method, you need an access key that is authorized for all of these Shinami products:
- Wallet Services
- Gas Station
- Node Service
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet. |
sessionToken | string | The token generated by shinami_key_createSession with the same secret you used when creating this wallet. |
options | object | <TransactionBlockResponseOptions> - Optional. Sui options for specifying the transaction content to be returned |
requestType (deprecated) | string | <ExecuteTransactionRequestType> - Optional. The execution request type (WaitForEffectsCert or WaitForLocalExecution ). Note that calling this method - via our SDK or otherwise - does not have the same result as using the Mysten SDK v 1.6 and above for executeTransactionBlock in that it does not also call waitForTransaction to poll the Fullnode to ensure that the transaction has been indexed after execution. If you require read-after-write consistency you will need to explicitly call waitForTransaction after calling this method. |
SDK-only: tx | GaslessTransaction | TransactionKind and additional optional data sender , gasBudget , and gasPrice . The result of a call to buildGaslessTransaction . |
cURL only: txBytes | string | Base64 encoded TransactionKind (as opposed to TransactionData ) bytes. So, it does not include gas information. |
cURL only: gasBudget | string | (Optional) The gas budget you wish to use for the transaction, in MIST. The transaction will fail if the gas cost exceeds this value. - If provided, we use the value as the budget of the sponsorship. - If omitted, we estimate the transaction cost for you. We then add a buffer (5% for non-shared objects, 25% for shared objects) and use that total value as the budget of the sponsorship. |
Auto-budgeting notes
- As a part of auto-budgeting, we put your
transactionBytes
through a sui_dryRunTransactionBlock request as a free service before we attempt to sponsor it. This call will generate error messages for certain invalid transactions, such as if thetransactionBytes
are transferring an object that's not owned by thesender
address you provide. We'll return these errors back to you, which should be the same as if you had made asui_dryRunTransactionBlock
request yourself. We do not do this step if you manually budget, so any issues that would be caught bysui_dryRunTransactionBlock
will instead produce an error when you try to execute the transaction. - In the time between sponsorship and execution, shared objects can change in a way that increases their transaction cost. Therefore, we encourage you to execute sponsored transactions quickly, if possible, to ensure that the sponsorship amount is sufficient. This is why we add a larger buffer on auto-budgeted sponsorships when a shared object is involved. While we believe this buffer will work in most cases, we encourage you to monitor the success rate of your auto-budgeted transactions to gauge whether your specific use-case requires manually setting an even larger
gasBudget
.
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{allServicesAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_wal_executeGaslessTransactionBlock",
"params": [
"{{walletId}}",
"{{sessionToken}}",
"{{txBytes}}",
"{{gasBudget}}",
{
"showInput": false,
"showRawInput": false,
"showEffects": false,
"showEvents": false,
"showObjectChanges": false,
"showBalanceChanges": false
},
"{{requestType}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{allServicesAccessKey}});
await walletClient.executeGaslessTransaction(
{{walletId}},
{{sessionToken}},
{{tx}}, // if tx.gasBudget is undefined we use our auto-budgeting featur
{
showInput: false,
showRawInput: false,
showEffects: false,
showEvents: false,
showObjectChanges: false,
showBalanceChanges: false
},
{{requestType}} // must set to `None` or `WaitForLocalExecution` if showEffects, showObjectChanges, or showBalanceChanges are set to true
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.executeGaslessTransaction(
{{tx}}, // if tx.gasBudget is undefined we use our auto-budgeting feature
{
showInput: false,
showRawInput: false,
showEffects: false,
showEvents: false,
showObjectChanges: false,
showBalanceChanges: false
},
{{requestType}} // must set to `None` or `WaitForLocalExecution` if showEffects, showObjectChanges, or showBalanceChanges are set to true
);
Example Response
{
"jsonrpc": "2.0",
"result": {
"digest": "Em4C8d6rRSUQ72kUWd627UfXTqDAVWQjJq9tmUFfnrmm",
"confirmedLocalExecution": true
},
"id": 1
}
{
digest: 'B6j8ePkw84R1rpUqxmjaZ3dTuu6GhPdY9MqoSso6kAn7',
confirmedLocalExecution: true
}
{
digest: 'B6j8ePkw84R1rpUqxmjaZ3dTuu6GhPdY9MqoSso6kAn7',
confirmedLocalExecution: true
}
Response Fields
Type | Description |
---|---|
SuiTransactionBlockResponse | <SuiTransactionBlockResponse> containing information about the executed transaction. |
shinami_walx_setBeneficiary
Beneficiary Graph API
This API interacts with the Account Graph Move package.
Description
Designates a beneficiary account for this wallet in the specified beneficiary graph instance. Calling this method multiple times will override the previous designations.
Apps participating in Bullshark Quests can use this method to link up Shinami Invisible Wallets with their users' self-custody wallets. This way, the user's self-custody wallet (the beneficiary, containing a Bullshark NFT) will get credit for actions done by the associated Invisible Wallet. This method accepts any value that is a possible Sui Address someone could own. It does not guarantee that anyone actually owns a keypair for it and can sign transactions from it (because it does not require the beneficiary address owner to sign a transaction).
This method will use your Shinami Gas Station fund to pay for the transaction. It sponsors the transaction using the Gas Station fund tied to the access key used to make the request. To see how to set up an Access Key with rights to all services, see our Authentication and API Keys guide. Note that this call produces a Node service sui_executeTransactionBlock
request which counts against your daily request total (and so your billing).
We explicitly wait for the transaction to have been check-pointed and indexed by the Fullnode before returning a response to you to ensure read-after-write consistency.
To call this method, you need an access key that is authorized for all of these Shinami products:
- Wallet Services (for signing the transaction)
- Gas Station (for sponsoring the transaction)
- Node Service (for executing the transaction)
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated invisible wallet whose beneficiary you're setting. |
sessionToken | string | The token generated by shinami_key_createSession with the same secret you used when creating this wallet. |
beneficiaryGraphId | string | <ObjectID> - Id of the beneficiary graph instance. |
beneficiaryAddress | string | <SuiAddress> - Address of the beneficiary account. |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{allServicesAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_walx_setBeneficiary",
"params": [
"{{walletId}}",
"{{sessionToken}}",
"{{beneficiaryGraphId}}",
"{{beneficiaryAddress}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{allServicesAccessKey}});
await walletClient.setBeneficiary(
{{walletId}},
{{sessionToken}},
{{beneficiaryGraphId}},
{{beneficiaryAddress}}
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.setBeneficiary(
{{beneficiaryGraphId}},
{{beneficiaryAddress}}
);
Example Response
{
"jsonrpc":"2.0",
"result":"43i3D8vhQaJBhDGd1sdQY346w4HjTb79EPv2faNmoSGA",
"id":1
}
"5J671ff8U9CHAABek3PvYDKpYqFcZAVzHJPjp8Nyj3Mp"
"5J671ff8U9CHAABek3PvYDKpYqFcZAVzHJPjp8Nyj3Mp"
Response Data
Type | Description |
---|---|
string | <TransactionDigest> - Transaction digest for this operation. |
shinami_walx_unsetBeneficiary
Beneficiary Graph API
This API interacts with the Account Graph Move package.
Description
Clears any beneficiary designation for this wallet in the specified beneficiary graph instance.
This method will use your Shinami Gas Station fund to pay for the transaction. It sponsors the transaction using the Gas Station fund tied to the access key used to make the request. To see how to set up an Access Key with rights to all services, see our Authentication and API Keys guide. Note that this call produces a Node service sui_executeTransactionBlock
request which counts against your daily request total (and so your billing).
We explicitly wait for the transaction to have been check-pointed and indexed by the Fullnode before returning a response to you to ensure read-after-write consistency.
To call this method, you need an access key that is authorized for all of these Shinami products:
- Wallet Services
- Gas Station
- Node Service
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet whose beneficiary you're unsetting. |
sessionToken | string | The token generated by shinami_key_createSession with the same secret you used when creating this wallet. |
beneficiaryGraphId | string | <ObjectID> - Id of the beneficiary graph instance. |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {{allServicesAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_walx_unsetBeneficiary",
"params": [
"{{walletId}}",
"{{sessionToken}}",
"{{beneficiaryGraphId}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{allServicesAccessKey}});
await walletClient.unsetBeneficiary(
{{walletId}},
{{sessionToken}},
{{beneficiaryGraphId}}
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.unsetBeneficiary(
{{beneficiaryGraphId}}
);
Example Response
{
"jsonrpc":"2.0",
"result":"7Ha6scuitsHKETDvShoBa1axv3qdGaspfxFjYdBMDDAQ",
"id":1
}
"5fsyXsmutHWsSnVVqrsNNLnaWRk84pYiwHHhHMZiX4Ea"
"5fsyXsmutHWsSnVVqrsNNLnaWRk84pYiwHHhHMZiX4Ea"
Response Data
Type | Description |
---|---|
string | <TransactionDigest> - Transaction digest for this operation |
shinami_walx_getBeneficiary
Beneficiary Graph API
This API interacts with the Account Graph Move package.
Description
Gets the beneficiary designation for this wallet in the specified beneficiary graph instance. This is a convenience method on top of suix_getDynamicFieldObject
.
To call this method, you need an access key that is authorized for all of these Shinami products:
- Wallet Services
- Node Service
Request Parameters
Name | Type | Description |
---|---|---|
walletId | string | Your unique, internal id for the associated Invisible Wallet whose beneficiary you're asking for. |
beneficiaryGraphId | string | <ObjectID> - Id of the beneficiary graph instance |
Example Request Template
The TypeScript example uses the Shinami Clients SDK.
Replace all instances of {{name}}
with the actual value for that name
curl https://api.shinami.com/sui/wallet/v1 \
-X POST \
-H 'X-API-Key: {walletAndNodeServicesAccessKey}}' \
-H 'Content-Type: application/json' \
-d '{
"jsonrpc": "2.0",
"method": "shinami_walx_getBeneficiary",
"params": [
"{{walletId}}",
"{{beneficiaryGraphId}}"
],
"id": 1
}'
import { WalletClient } from "@shinami/clients/sui";
const walletClient = new WalletClient({{walletAndNodeServicesAccessKey}});
await walletClient.getBeneficiary(
{{walletId}},
{{beneficiaryGraphId}}
);
import {
WalletClient,
KeyClient,
ShinamiWalletSigner
} from "@shinami/clients/sui";
const keyClient = new KeyClient({{walletAccessKey}});
const walletClient = new WalletClient({{walletAccessKey}});
const signer = new ShinamiWalletSigner(
{{walletId}},
walletClient,
{{walletSecret}},
keyClient
);
await signer.getBeneficiary(
{{beneficiaryGraphId}}
);
Example Response
// The wallet has a beneficiary in the provided beneficiary graph
{
"jsonrpc":"2.0",
"result":"0xa39edfb89e6a21e89570711845c6c8f412d86bb208e571faf6ea1fc6470172d7",
"id":1
}
// The wallet does not have a beneficiary in the provided beneficiary graph
{
"jsonrpc":"2.0",
"result":null,
"id":1
}
// The wallet has a beneficiary in the provided beneficiary graph
"0xa39edfb89e6a21e89570711845c6c8f412d86bb208e571faf6ea1fc6470172d7"
// The wallet does not have a beneficiary in the provided beneficiary graph
null
// The wallet has a beneficiary in the provided beneficiary graph
"0xa39edfb89e6a21e89570711845c6c8f412d86bb208e571faf6ea1fc6470172d7"
// The wallet does not have a beneficiary in the provided beneficiary graph
null
Response Data
Type | Move Type | Description |
---|---|---|
string || null | address | The network-agnostic Sui address of the Invisible Wallet created for this walletId. Null if no beneficiary is designated. |